Someone outside of the forums ask me if it was possible to have a 'modal' pop up window that allows the user to enter data. Turns out, this can be done. There are two ways I found to do it:
(Note: code samples are in C# but this should work fine in VB.NET)
1. Short & sweet
The simplest way to do this is first to include a client-side DLL & then include it:
//<ref>Microsoft.VisualBasic.dll</Ref>
using Microsoft.VisualBasic;
Then, you can have a method similar to this:
public void SimplePopUp()
{
ThisForm.Components["edit1_SITE"].SetValue(Interaction.InputBox("Tell me something","TYPE NOW!",""));
}
This will pop up a predefined window that allows text to be entered & returns a string:
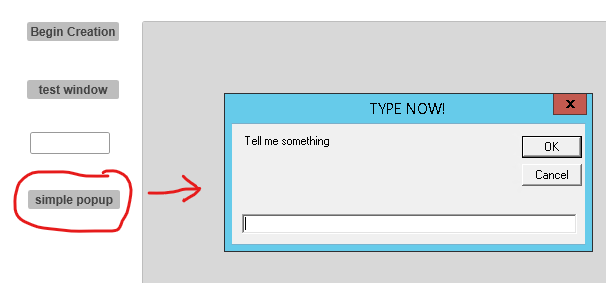
2. 'Build from scratch'
It is also possible to design your own pop up with multiple values. For this to work, it requires a second external DLL to be linked:
//<Ref>System.Windows.Forms.dll</Ref>
//<Ref>Microsoft.VisualBasic.dll</Ref>
using System.Windows.Forms;
using Microsoft.VisualBasic;
You can then build your own form (by hand, sadly) using code. I took the example found here: https://www.delftstack.com/howto/csharp/create-an-input-dialog-box-in-csharp/
Then, you simple call the method:
public void GetWindowInfo()
{
string input = "...";
//Display the custom input dialog box with the following prompt, window title, and dimensions
ShowInputDialogBox(ref input, "What is at the end of the rainbow?", "Riddle", 300, 200);
ThisForm.Components["edit1_SITE"].SetValue(input);
}
Note that in this solution, you need to pass variables by ref. You get a window that looks like this:
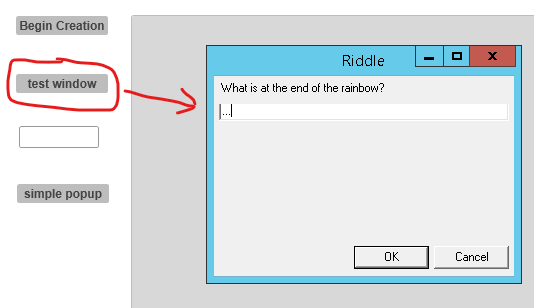
As these solutions require access to DLL's located on the client's computer, this may be a 'mileage may vary' solution.
Hope this is helpful to someone 
www.linkedin.com/.../