Looking for some guidance or code samples of how to send/receive an ION API call in Application Engine.
for bearer token (initial handshake) :
#define EngineVersion 5.0
#define RuntimeVersion 5.0
IONResponse_RequestToken()
{
//string tokenUrl = "
string clientId = "myclientid";
string clientSecret = "myclientSecret ";
string username = "myusername";
string password = "mypassword";
string serviceAccount = "my@email.com"; // service account email from .ionapi authorised app creation?
RequestMethodType methodType = RequestMethodType.Post; // POST for bearer token
string suite = "FSM"; // suite = api name from API Gateways? FSM, infor CSP, infor ION etc
string product = "https://mingle-sso.inforcloudsuite.com:443/mytenant_TST; // Product = tenant URL ?
string restPath = "/as/token.oauth2"; // Endpoint for token generation
IONRequestMessage request = IONCreateRequestMessage(serviceAccount, methodType, suite, product, restPath);
// Add request parameters
IONAddRequestParameter(RequestParameterType.Query, request, "grant_type", "password");
IONAddRequestParameter(RequestParameterType.Query, request, "client_id", clientId);
IONAddRequestParameter(RequestParameterType.Query, request, "client_secret", clientSecret);
IONAddRequestParameter(RequestParameterType.Query, request, "username", username);
IONAddRequestParameter(RequestParameterType.Query, request, "password", password);
IONAddRequestParameter(RequestParameterType.Header, request, "Content-Type", "application/x-www-form-urlencoded");
IONResponse response = IONSendRequestMessage(request);
return response;
}
We are trying to use IONRequestMessage to retrieve data from FSM business classes, not sure if I am filling the right context into suite, product and restPath variables. Assuming suite to be the api that we want to use, product as the unique tenant url and the restPath as the oAuth token url (which would then be traditionaly joined together.
We then moved on to actual API calls, a bearer token manually inserted for now, we make a call to the GeneralLedgerTotalList operation in the FSM api. Have tried many combinations of product and restpath, including FSM/fsm/soap/business class, not including business class etc. have also tried including GeneralLedgerTotal & GeneralLedgerTotalList as query parameters too.
Manually pulled a bearer token from postman to test this process too but returns the same error as the bearer request from application engine.
#define EngineVersion 5.0
#define RuntimeVersion 5.0
IONResponse_RequestGeneralLedgerTotalList()
{
string bearerToken = "Bearer eyJraWQiOiJ";
string serviceAccount = "my@email.com"; // The service account email
string suite = "FSM";
string product = "https://mingle-ionapi.inforcloudsuite.com/mytenant_TST; //tenant + api base url
string restPath = "/classes/GeneralLedgerTotal/lists/GeneralLedgerTotalList"; // Endpoint for api operation
IONRequestMessage request = IONCreateRequestMessage(serviceAccount, RequestMethodType.Get, suite, product, restPath);
// Add request parameters
IONAddRequestParameter(RequestParameterType.Header, request, "Authorization", bearerToken); //bearer token auth header
IONAddRequestParameter(RequestParameterType.Query, request, "_limit", "100"); //required parameter
IONResponse response = IONSendRequestMessage(request);
return response;
}
Not sure what we are doing wrong in these two calls, but assuming we are just putting incorrect/unexpected data into the IONRequestMessage paremeters. Client ID, service account username / password have all been validated and working in postman where we are able to get a bearer and pull data from the api.
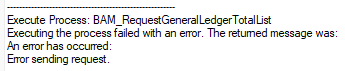
Bit more verbose when we throw in some watches but not more useful in terms of understanding the issue:
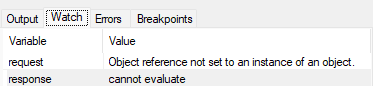