Hi,
I've created a custom form (an .ascx file) that launches into a dialog window from a button on the Opportunity details smartpart. Within this custom form, I have a GridView with editable rows. What I'm trying to achieve is to get the new values that were entered into each textbox to evaluate by clicking "update". This doesn't work. The values are always reverted back to the original, old values.
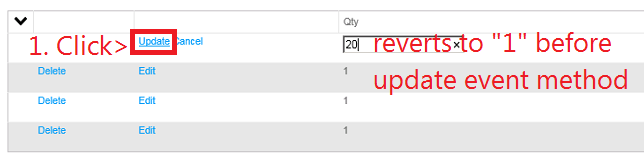
Looking into and debugging this issue, I realized a few things:
- The Page_Load event fires every time a textbox within the gridview is clicked (Edit, Update, lost focus). This causes the grid to re-bind to the sqlDataSource every time, which in turn reverts the values as described above.
2. Which I believe is the root cause, is that after debugging the Page_Load event, I see that Page.IsPostBack is ALWAYS true, meaning I cannot work out the logic to avoid rebinding the grid every time:
protected void Page_Load(object sender, EventArgs e) {
if (Visible) {
if (!this.IsPostBack) {
// currently never hits this point
}
//bind grids
BindProductsGrid();
BindOppProductsGrid();
...
I've tried several workarounds for this, such as creating an event handler for each gridview textbox control on RowDataBound, or storing the values in a session variable:
protected void grdOppProducts_RowDataBound(object sender, GridViewRowEventArgs e) {
if (e.Row.RowType == DataControlRowType.DataRow) {
TextBox txtQuantity = (TextBox)e.Row.FindControl("txtQuantity");
if(txtQuantity != null){
txtQuantity.TextChanged += new EventHandler(grdOppProducts_OnQtyChanged);
}
}
}
...but every click or focus change to the gridview or form causes a postback and the values are lost before I can intercept them.
What am I doing wrong? Why is Page.IsPostBack always true? I'm attempting to use the built-in gridview events (RowEditing, RowUpdating) so I'm assuming this should work in practice.
For reference, here's my gridview definition:
<saleslogix:slxgridview runat="server" id="oppProdgrdView" gridlines="None" AllowPaging="False" selectedrowstyle LightCyan="backcolor"
autogeneratecolumns="false"
autoGenerateEditButton="false"
autoGenerateDeleteButton="true"
OnRowDataBound="grdOppProducts_RowDataBound"
OnRowCommand="grdOppProducts_RowCommand"
OnRowUpdating="grdOppProducts_RowUpdating"
OnRowUpdated="grdOppProducts_RowUpdated"
OnRowCancelingEdit="grdOppProducts_RowCancelingEdit"
OnRowEditing="grdOppProducts_RowEditing"
OnRowDeleting="grdOppProducts_RowDeleting"
cellpadding="1" cssclass="datagrid" pagerstyle-cssclass="gridPager"
alternatingrowstyle-cssclass="rowdk" rowstyle-cssclass="rowlt" selectedrowstyle-cssclass="rowSelected" showemptytable="True" enableviewstate="false"
emptytablerowtext="<%$ resources:dgOppProducts.EmptyTableRowText %>" expandablerows="True" resizablecolumns="True" currentsortdirection="Ascending" currentsortexpression=""
datasourceid="SqlDataSource2" datakeynames="OPPPRODUCTID" showsorticon="False" useslxpagertemplate="True" AllowSorting="true" PageSize="16">
<Columns>
<asp:commandfield showeditbutton="true" causesvalidation="false" headertext=""/>
<asp:BoundField DataField="PRODUCTID" ItemStyle-CssClass="hidden-column" HeaderStyle-CssClass="hidden-column"
HeaderText="ProductId">
</asp:BoundField>
<asp:TemplateField HeaderText="Qty" HeaderStyle-HorizontalAlign="Left">
<EditItemTemplate>
<asp:TextBox ID="txtQuantity" runat="server" Text='<%# Bind("QUANTITY") %>'> OnTextChanged="grdOppProducts_OnQtyChanged" autoPostback="true"></asp:TextBox>
</EditItemTemplate>
<ItemTemplate>
<asp:Label ID="lblQuantity" runat="server" Text='<%# Bind("QUANTITY") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
</Columns>
<PagerStyle CssClass="gridPager"></PagerStyle>
<RowStyle CssClass="rowlt"></RowStyle>
<SelectedRowStyle CssClass="rowSelected"></SelectedRowStyle>
</saleslogix:slxgridview>